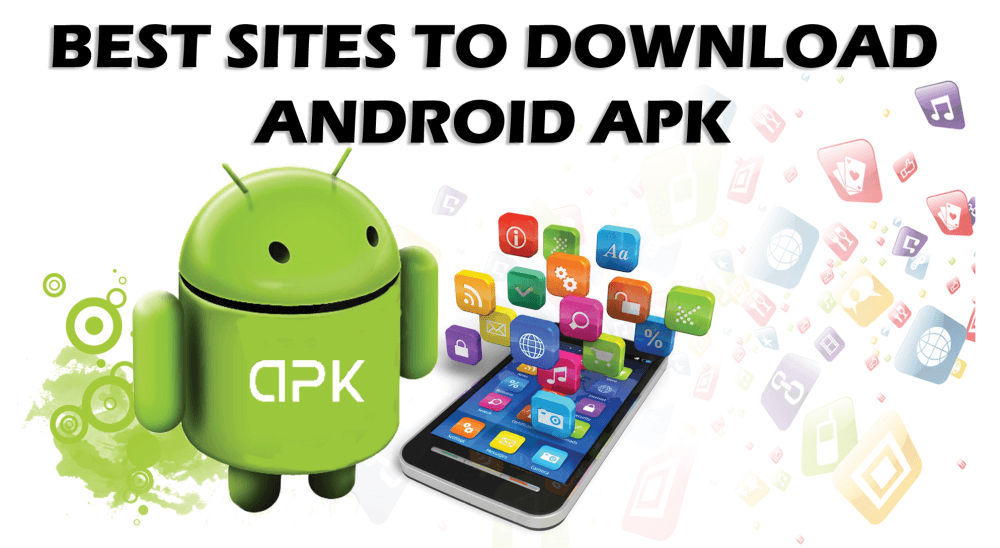
Android App Development
Android app development is a dynamic and fast-growing field that plays a crucial role in our tech-driven world. With billions of active Android devices globally, building apps for Android can lead to powerful opportunities for businesses, developers, and entrepreneurs alike. Whether you’re a beginner or looking to advance your skills, this guide provides an end-to-end overview of Android app development.
What is Android App Development?
Android app development is the process of creating applications that run on devices powered by the Android operating system. These apps can be anything from mobile games, productivity tools, or social media platforms to enterprise-level applications.
Key Platforms:
- Android smartphones and tablets
- Wear OS (smartwatches)
- Android TV
- Android Auto
Why Choose Android?
- Largest Market Share: Android holds the majority share of the global smartphone market.
- Open Source: Based on the Linux kernel, Android is open-source and highly customizable.
- Wide Device Range: Apps can run on a broad range of devices, from budget phones to high-end gadgets.
- Google Integration: Easy integration with Google services like Maps, Firebase, and Assistant.
Getting Started with Android Development
1. Learn the Basics of Programming
Before diving into Android, you should be comfortable with programming concepts. The primary languages used in Android development are:
- Java (traditional)
- Kotlin (modern, official Android language)
Kotlin is now the recommended language by Google for Android development due to its concise syntax and safety features.
2. Set Up the Development Environment
To start building Android apps, install the following tools:
- Android Studio: Official IDE for Android development.
- Java Development Kit (JDK): Required to run Java or Kotlin.
- Android SDK: A collection of tools and libraries necessary for Android development.
3. Understand Android Architecture
An Android app is built using components:
- Activities: Screens in your app.
- Fragments: Modular parts of a UI.
- Services: Background processes.
- Broadcast Receivers: Listen for system-wide events.
- Content Providers: Share data between apps.
Also, you’ll work with:
- XML for UI layout
- Gradle for build automation
Designing the UI
Android uses XML for defining UI layouts. You can create layouts in:
- XML files
- Jetpack Compose (modern declarative UI toolkit)
Material Design:
Follow Google’s Material Design Guidelines to build user-friendly and consistent UI experiences.
Building App Logic
Use Kotlin or Java to handle logic, events, user input, and interactions. Android Studio provides tools like:
- Live templates
- Code completion
- Debugging tools
Data Storage and Management
You can store app data using:
- SharedPreferences (key-value pairs)
- Room Database (SQLite ORM for local database)
- Cloud Databases like Firebase Realtime Database or Firestore
Networking in Android
Fetch data from the internet using:
- Retrofit: Type-safe HTTP client
- Volley: Google’s library for networking
- OkHttp: Efficient network library
Advanced Concepts
1. Jetpack Libraries
Jetpack is a set of libraries, tools, and architectural guidance for building robust Android apps.
Key Jetpack components:
- LiveData
- ViewModel
- Navigation Component
- DataBinding
- WorkManager
2. Dependency Injection
Helps manage complex dependencies using libraries like:
- Dagger
- Hilt
- Koin
3. Asynchronous Programming
Use Coroutines (in Kotlin) or RxJava for background tasks and asynchronous operations.
Testing Android Apps
Ensure your app works well using:
- Unit Tests (JUnit)
- UI Tests (Espresso)
- Mocking (Mockito)
Publishing to Google Play Store
Steps to release your app:
- Create a Google Play Console account.
- Prepare app assets (icons, screenshots, descriptions).
- Build a signed APK or App Bundle.
- Submit for review and publish.
Monetization Strategies
Monetize your Android app through:
- In-app purchases
- Advertisements (e.g., AdMob)
- Subscription models
- Freemium models
Tools and Resources
- Android Developer Docs: developer.android.com
- Kotlin Lang: kotlinlang.org
- Firebase: Backend-as-a-Service by Google
- GitHub: For version control and open-source projects
Conclusion
Android app development is a rewarding skill with limitless potential. With powerful tools, a massive user base, and a supportive community, it’s a great platform for building anything from hobby projects to large-scale applications. Whether you’re a solo developer or part of a team, mastering Android can open many doors in the tech industry.
